- Python on the Web. It doesn't look like GC is the only thing that stops Python code from targeting WebAssembly/asm.js. Both represent low-level statically typed code, in which Python code can't (realistically) be represented.
- The Web Server Gateway Interface (or “WSGI” for short) is a standard interface between web servers and Python web application frameworks. By standardizing behavior and communication between web servers and Python web frameworks, WSGI makes it possible to write portable Python web code that can be deployed in any WSGI-compliant web server.
Source code:Lib/http/server.py
This module defines classes for implementing HTTP servers (Web servers).
Warning
Despite the hype around machine learning, Python has done enough to conquer the web application domain as well. # on running python app.py app.run # run the flask app Running the Application.
http.server
is not recommended for production. It only implementsbasic security checks.
One class, HTTPServer
, is a socketserver.TCPServer
subclass.It creates and listens at the HTTP socket, dispatching the requests to ahandler. Code to create and run the server looks like this:
http.server.
HTTPServer
(server_address, RequestHandlerClass)¶This class builds on the TCPServer
class by storingthe server address as instance variables named server_name
andserver_port
. The server is accessible by the handler, typicallythrough the handler’s server
instance variable.
http.server.
ThreadingHTTPServer
(server_address, RequestHandlerClass)¶This class is identical to HTTPServer but uses threads to handlerequests by using the ThreadingMixIn
. Thisis useful to handle web browsers pre-opening sockets, on whichHTTPServer
would wait indefinitely.
New in version 3.7.
The HTTPServer
and ThreadingHTTPServer
must be givena RequestHandlerClass on instantiation, of which this moduleprovides three different variants:
http.server.
BaseHTTPRequestHandler
(request, client_address, server)¶This class is used to handle the HTTP requests that arrive at the server. Byitself, it cannot respond to any actual HTTP requests; it must be subclassedto handle each request method (e.g. GET or POST).BaseHTTPRequestHandler
provides a number of class and instancevariables, and methods for use by subclasses.
The handler will parse the request and the headers, then call a methodspecific to the request type. The method name is constructed from therequest. For example, for the request method SPAM
, the do_SPAM()
method will be called with no arguments. All of the relevant information isstored in instance variables of the handler. Subclasses should not need tooverride or extend the __init__()
method.
BaseHTTPRequestHandler
has the following instance variables:
client_address
¶Contains a tuple of the form (host,port)
referring to the client’saddress.
server
¶Contains the server instance.
close_connection
¶Boolean that should be set before handle_one_request()
returns,indicating if another request may be expected, or if the connection shouldbe shut down. Download anime green green batch 360p.
requestline
¶Contains the string representation of the HTTP request line. Theterminating CRLF is stripped. This attribute should be set byhandle_one_request()
. If no valid request line was processed, itshould be set to the empty string.
command
¶Contains the command (request type). For example, 'GET'
.
path
¶Contains the request path. If query component of the URL is present,then path
includes the query. Using the terminology of RFC 3986,path
here includes hier-part
and the query
.
request_version
¶Contains the version string from the request. For example, 'HTTP/1.0'
.
headers
¶Holds an instance of the class specified by the MessageClass
classvariable. This instance parses and manages the headers in the HTTPrequest. The parse_headers()
function fromhttp.client
is used to parse the headers and it requires that theHTTP request provide a valid RFC 2822 style header.
rfile
¶An io.BufferedIOBase
input stream, ready to read fromthe start of the optional input data.
wfile
¶Contains the output stream for writing a response back to theclient. Proper adherence to the HTTP protocol must be used when writing tothis stream in order to achieve successful interoperation with HTTPclients.
Changed in version 3.6: This is an io.BufferedIOBase
stream.

BaseHTTPRequestHandler
has the following attributes:
server_version
¶Specifies the server software version. You may want to override this. Theformat is multiple whitespace-separated strings, where each string is ofthe form name[/version]. For example, 'BaseHTTP/0.2'
.
sys_version
¶Contains the Python system version, in a form usable by theversion_string
method and the server_version
classvariable. For example, 'Python/1.4'
.
error_message_format
¶Specifies a format string that should be used by send_error()
methodfor building an error response to the client. The string is filled bydefault with variables from responses
based on the status codethat passed to send_error()
.
error_content_type
¶Specifies the Content-Type HTTP header of error responses sent to theclient. The default value is 'text/html'
.
protocol_version
¶This specifies the HTTP protocol version used in responses. If set to'HTTP/1.1'
, the server will permit HTTP persistent connections;however, your server must then include an accurate Content-Length
header (using send_header()
) in all of its responses to clients.For backwards compatibility, the setting defaults to 'HTTP/1.0'
.
MessageClass
¶Specifies an email.message.Message
-like class to parse HTTPheaders. Typically, this is not overridden, and it defaults tohttp.client.HTTPMessage
.
responses
¶This attribute contains a mapping of error code integers to two-element tuplescontaining a short and long message. For example, {code:(shortmessage,longmessage)}
. The shortmessage is usually used as the message key in anerror response, and longmessage as the explain key. It is used bysend_response_only()
and send_error()
methods.
A BaseHTTPRequestHandler
instance has the following methods:
handle
()¶Calls handle_one_request()
once (or, if persistent connections areenabled, multiple times) to handle incoming HTTP requests. You shouldnever need to override it; instead, implement appropriate do_*()
methods.
handle_one_request
()¶This method will parse and dispatch the request to the appropriatedo_*()
method. You should never need to override it.
handle_expect_100
()¶When a HTTP/1.1 compliant server receives an Expect:100-continue
request header it responds back with a 100Continue
followed by 200OK
headers.This method can be overridden to raise an error if the server does notwant the client to continue. For e.g. server can choose to send 417ExpectationFailed
as a response header and returnFalse
.
send_error
(code, message=None, explain=None)¶Sends and logs a complete error reply to the client. The numeric codespecifies the HTTP error code, with message as an optional, short, humanreadable description of the error. The explain argument can be used toprovide more detailed information about the error; it will be formattedusing the error_message_format
attribute and emitted, aftera complete set of headers, as the response body. The responses
attribute holds the default values for message and explain thatwill be used if no value is provided; for unknown codes the default valuefor both is the string ???
. The body will be empty if the method isHEAD or the response code is one of the following: 1xx
,204NoContent
, 205ResetContent
, 304NotModified
.
Changed in version 3.4: The error response includes a Content-Length header.Added the explain argument.
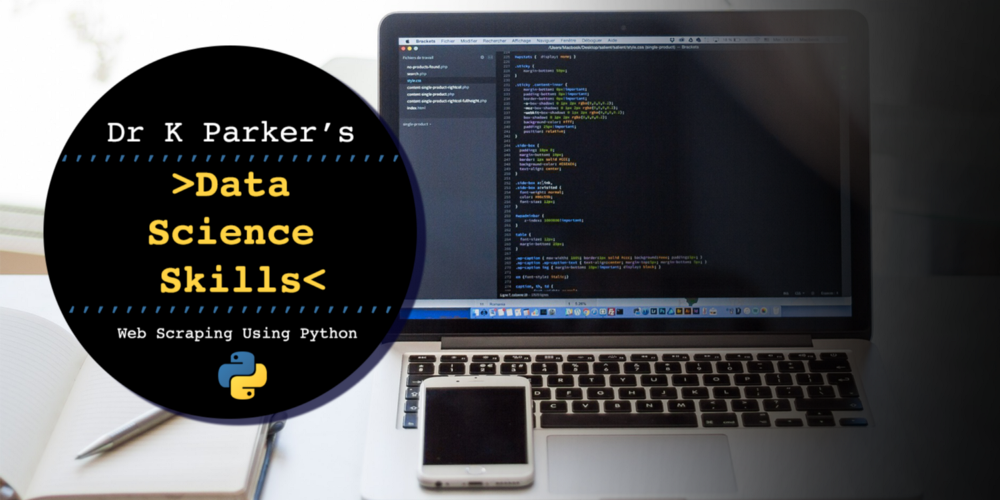
send_response
(code, message=None)¶Adds a response header to the headers buffer and logs the acceptedrequest. The HTTP response line is written to the internal buffer,followed by Server and Date headers. The values for these two headersare picked up from the version_string()
anddate_time_string()
methods, respectively. If the server does notintend to send any other headers using the send_header()
method,then send_response()
should be followed by an end_headers()
call.
Changed in version 3.3: Headers are stored to an internal buffer and end_headers()
needs to be called explicitly.
send_header
(keyword, value)¶Adds the HTTP header to an internal buffer which will be written to theoutput stream when either end_headers()
or flush_headers()
isinvoked. keyword should specify the header keyword, with valuespecifying its value. Note that, after the send_header calls are done,end_headers()
MUST BE called in order to complete the operation.
Changed in version 3.2: Headers are stored in an internal buffer.
send_response_only
(code, message=None)¶Sends the response header only, used for the purposes when 100Continue
response is sent by the server to the client. The headers notbuffered and sent directly the output stream.If the message is notspecified, the HTTP message corresponding the response code is sent.
end_headers
()¶Adds a blank line(indicating the end of the HTTP headers in the response)to the headers buffer and calls flush_headers()
.
Changed in version 3.2: The buffered headers are written to the output stream.
flush_headers
()¶Finally send the headers to the output stream and flush the internalheaders buffer.
log_request
(code='-', size='-')¶Logs an accepted (successful) request. code should specify the numericHTTP code associated with the response. If a size of the response isavailable, then it should be passed as the size parameter.
log_error
(..)¶Logs an error when a request cannot be fulfilled. By default, it passesthe message to log_message()
, so it takes the same arguments(format and additional values).
log_message
(format, ..)¶Logs an arbitrary message to sys.stderr
. This is typically overriddento create custom error logging mechanisms. The format argument is astandard printf-style format string, where the additional arguments tolog_message()
are applied as inputs to the formatting. The clientip address and current date and time are prefixed to every message logged.
version_string
()¶Returns the server software’s version string. This is a combination of theserver_version
and sys_version
attributes.
date_time_string
(timestamp=None)¶Returns the date and time given by timestamp (which must be None
or inthe format returned by time.time()
), formatted for a messageheader. If timestamp is omitted, it uses the current date and time.
The result looks like 'Sun,06Nov199408:49:37GMT'
.
log_date_time_string
()¶Returns the current date and time, formatted for logging.
address_string
()¶Returns the client address.
Changed in version 3.3: Previously, a name lookup was performed. To avoid name resolutiondelays, it now always returns the IP address.
http.server.
SimpleHTTPRequestHandler
(request, client_address, server, directory=None)¶This class serves files from the directory directory and below,or the current directory if directory is not provided, directlymapping the directory structure to HTTP requests.
Changed in version 3.9: The directory parameter accepts a path-like object.
A lot of the work, such as parsing the request, is done by the base classBaseHTTPRequestHandler
. This class implements the do_GET()
and do_HEAD()
functions.
The following are defined as class-level attributes ofSimpleHTTPRequestHandler
:
server_version
¶This will be 'SimpleHTTP/'+__version__
, where __version__
isdefined at the module level.
extensions_map
¶A dictionary mapping suffixes into MIME types, contains custom overridesfor the default system mappings. The mapping is used case-insensitively,and so should contain only lower-cased keys.
Changed in version 3.9: This dictionary is no longer filled with the default system mappings,but only contains overrides.
The SimpleHTTPRequestHandler
class defines the following methods:
do_HEAD
()¶This method serves the 'HEAD'
request type: it sends the headers itwould send for the equivalent GET
request. See the do_GET()
method for a more complete explanation of the possible headers.
do_GET
()¶The request is mapped to a local file by interpreting the request as apath relative to the current working directory.
If the request was mapped to a directory, the directory is checked for afile named index.html
or index.htm
(in that order). If found, thefile’s contents are returned; otherwise a directory listing is generatedby calling the list_directory()
method. This method usesos.listdir()
to scan the directory, and returns a 404
errorresponse if the listdir()
fails.
If the request was mapped to a file, it is opened. Any OSError
exception in opening the requested file is mapped to a 404
,'Filenotfound'
error. If there was a 'If-Modified-Since'
header in the request, and the file was not modified after this time,a 304
, 'NotModified'
response is sent. Otherwise, the contenttype is guessed by calling the guess_type()
method, which in turnuses the extensions_map variable, and the file contents are returned.
A 'Content-type:'
header with the guessed content type is output,followed by a 'Content-Length:'
header with the file’s size and a'Last-Modified:'
header with the file’s modification time.
Then follows a blank line signifying the end of the headers, and then thecontents of the file are output. If the file’s MIME type starts withtext/
the file is opened in text mode; otherwise binary mode is used.
For example usage, see the implementation of the test()
functioninvocation in the http.server
module.
Changed in version 3.7: Support of the 'If-Modified-Since'
header.
The SimpleHTTPRequestHandler
class can be used in the followingmanner in order to create a very basic webserver serving files relative tothe current directory:
http.server
can also be invoked directly using the -m
switch of the interpreter with a portnumber
argument. Similar tothe previous example, this serves files relative to the current directory:
By default, server binds itself to all interfaces. The option -b/--bind
specifies a specific address to which it should bind. Both IPv4 and IPv6addresses are supported. For example, the following command causes the serverto bind to localhost only:
New in version 3.4: --bind
argument was introduced.
New in version 3.8: --bind
argument enhanced to support IPv6
By default, server uses the current directory. The option -d/--directory
specifies a directory to which it should serve the files. For example,the following command uses a specific directory:
New in version 3.7: --directory
specify alternate directory
http.server.
CGIHTTPRequestHandler
(request, client_address, server)¶This class is used to serve either files or output of CGI scripts from thecurrent directory and below. Note that mapping HTTP hierarchic structure tolocal directory structure is exactly as in SimpleHTTPRequestHandler
.
Note
CGI scripts run by the CGIHTTPRequestHandler
class cannot executeredirects (HTTP code 302), because code 200 (script output follows) issent prior to execution of the CGI script. This pre-empts the statuscode.
The class will however, run the CGI script, instead of serving it as a file,if it guesses it to be a CGI script. Only directory-based CGI are used —the other common server configuration is to treat special extensions asdenoting CGI scripts.
The do_GET()
and do_HEAD()
functions are modified to run CGI scriptsand serve the output, instead of serving files, if the request leads tosomewhere below the cgi_directories
path.
The CGIHTTPRequestHandler
defines the following data member:
cgi_directories
¶This defaults to ['/cgi-bin','/htbin']
and describes directories totreat as containing CGI scripts.
The CGIHTTPRequestHandler
defines the following method:

do_POST
()¶Run Python
This method serves the 'POST'
request type, only allowed for CGIscripts. Error 501, “Can only POST to CGI scripts”, is output when tryingto POST to a non-CGI url.
Note that CGI scripts will be run with UID of user nobody, for securityreasons. Problems with the CGI script will be translated to error 403.
Code Runner Python
CGIHTTPRequestHandler
can be enabled in the command line by passingthe --cgi
option: